Overview
In the current tutorial, we will get to know what is fetchMode, how it is used to fetch the entity and an introduction to @org.hibernate.annotations.Fetch annotation.
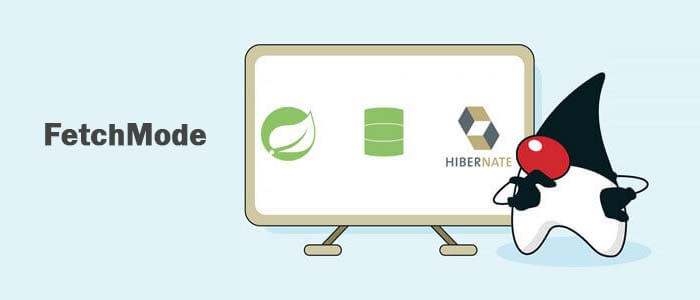
Setting up the Example
As an example, we will use a Customer entity with two properties, an id and a set of orders.
Next, we will create the Orders entity, consisting of the Customer entity as a reference.
Next using the test class, we will understand the usage of @Fetch annotation.
From fetching the customer details from the database, we are using Hibernate session.
Below is the code to get customer details along with orders from the database.
But there can be different ways to fetch the orders from the database, either we can join with the Customer entity to get the details or generate a single query for we can use another query to get all the order details.
1) FetchMode.SELECT
it is the default strategy, it will use for fetching dependent lazily.
Fetch is used to describe how to hibernate should retrieve the dependent property when we lookup for a parent entity.
Using SELECT Hibernate will fetch orders entity lazily.
For suppose we want to fetch all the customers and their orders the code will look like the below:
This will result in the below-generated SQL statements.
For each customer it will fetch the orders using an individual query, this will result in a very well-known n+1 select problem. The first query is used to fetch the customers, and n queries are used for fetching each customer.
2) @BatchSize
Fetch mode is an optional configuration to specify the batch size, instead of retrieving each customer order separately, Hibernate will fetch orders using batches. Using @BatchSize we can configure the batch size. This way we can decrease the number of queries required to fetch the orders.
3) FetchMode.JOIN
It is almost the same eager loading, the orders and customer entities are queries using the same query.
ALSO READ
In this case, only a single query does execute for all required entities.
Hibernate generated SQL statement will look like this:
4) FetchMode.SUBSELECT
it is used only if the nested property is a type of collection.
Using this fetchmode, only 2 queries will execute, one for fetching the customers, and one more query for fetching all customer orders.
And the Hibernate Generated queries look like below:
FetchMode vs. FetchType
In general, FetchMode defines how Hibernate will fetch the data (by select, join or subselect). FetchType, on the other hand, defines whether Hibernate will load data eagerly or lazily.
The exact rules between these two are as follows:
- 1) if the code doesn’t set FetchMode, the default one is JOIN, and FetchType works as defined
- 2) with FetchMode.SELECT or FetchMode.SUBSELECT set, FetchType also works as defined
- 3) With FetchMode.JOIN set, FetchType is ignored and a query is always eager
You should keep some points in mind when you hire Java developers such as they should be able to solve problems.
Conclusion
In this tutorial, we learned about what FetchMode is, how to use Hibernate internally to fetch entity mappings, and different types of FetchModes and their usage. Finally, we understood the differences between Fetch Mode and FetchType.
Source code: Hibenet Example Code