Overview
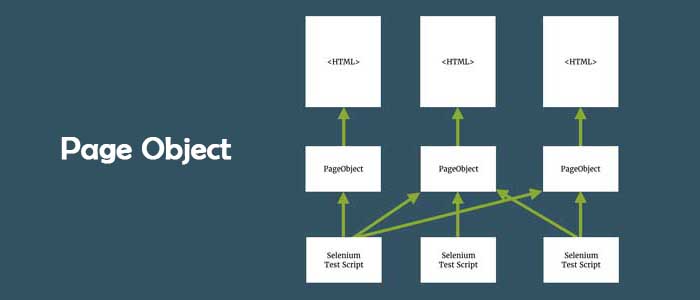
As the name suggests, the Page object Model is a design pattern but not a framework. There’s a myth in the testing community that Page Object Model is a framework integrated with Selenium. However, it is a design pattern that provides a way to create an object repository of all web UI elements that would be used in creating an automation framework for our web application.
In-Page Object Model, we will be working on Pages, and the “Page” refers to Java classes, which would be storing web page corresponding locators and methods to perform operations on those web pages.
When we are working on a small test automation project, using Page Object Model doesn’t matter. However, when we are working on test automation for a large growing project, it is highly recommended to use Page Object Model. So for that, our test suite typically grows with time. Hence it would become difficult to maintain those test scripts if we are not following the Page Object Model.
Example: We have 20 scripts in our test suite for 20 different test cases, but they do have common web elements interaction. Any change in these elements will force us to change the locators in all 20 scripts, however, this would be time-consuming and challenging to maintain. Hence, it is recommended to use Page Object Model, where we can store our locators in one single place so that they don’t consume time when any changes are required.
Advantages of using the Page Object Model
- Helps in easy maintenance of the test suite.
- Develops scripts in a more readable format.
- Makes scripts reusable.
- Efficient and reliable.
Disadvantages of using the Page Object Model
- It requires initial high efforts and time to set up the model.
- Requires technical-sounding testers with functional programming practices.
Sample code of Object Repository
Code Walkthrough
The above code refers to the object repository where we have stored our web UI locators for our different test cases. For this, we have used Selenium predefined class “By” to contain web Elements. In short, we have created a function using the defined locators that would act like a bunch of operations performing on a web application.
Sample Test Cases using the above Object Repository
Code Walkthrough
In the above code, we have created two test cases. We have used BeforeMethod annotation to set up the browser configuration and AfterMethod annotation to quit the browser after the execution of each test case. In both the test cases, there are few common web operations; hence, using Page Object Model in such instances becomes necessary else it would lead to code redundancy. In both the test cases, we have called out the function that we have created in an Object Repository class. If any of the locators are to be changed in the future, we can make a quick change in Object Repository class in no time.
Page Object Model with Page Factory
Page Factory Class is another way of implementing the Page Object Model. Even here, our focus is to maintain separate classes for our locators and test cases. In this, we make use of @FindBy annotation to find our web elements. To initialize web elements, Page Factory class provides a method called initElements used along with parameters i.e., WebDriver reference and class name storing the web locators.
@FindBy annotation can accept any Selenium locator as a property.
Sample Code for Object Repository using Page Factory Class
Code Walkthrough
The above code refers to the Object Repository of our test cases. The code is more or less similar to the one we have used earlier with the “By” class. The difference that is to be noticed is only that we have used the @FindBy annotation to find the web elements. With each FindBy annotation, we have also used a WebElement interface reference to store the located element. As well, To initialize the web elements located using @FindBy, we have used PageFactory class with its predefined method entitlements.
Sample Test Cases using the above Object Repository
Code Walkthrough
In the above code, we have created two test cases similar to the one created in the standard approach of the Page Object Model. As mentioned above, the difference between both methods comes only in Page Object Repository which means the way we want to organize web locators in our Page class.
Summarizing it all!!
The POM with Selenium is classified into two approaches. The first one is the standard approach in which we used the “BY” class to store our locators. Another one is the page factory approach in which we use the @FindBy annotation to find and store locators. The purpose of both methods is the same that is to create separate class files for web UI locators and test cases. Page Object Model plays a vital role in Test Automation of large growing projects in any Offshore Software Testing Company. So guys, give it a try, this is very useful in Selenium-based automation projects.