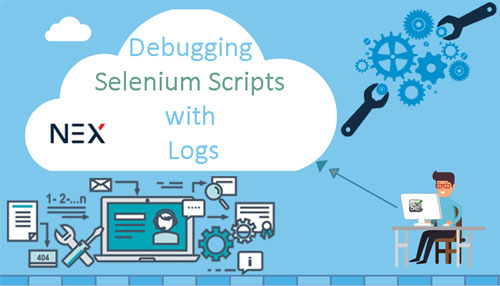
Whenever you are maintaining automated Selenium tests, it is very important to have a logging framework which makes debugging very easy. You can’t find the failures with console output command in Java. You should have proper logs so that you can easily debug the selenium scripts with ease. Now, you would be imagining why we actually require logging framework. So, a good logging framework will give us a good understanding of tests suite execution. You can store the logs in third-party files that can be shared easily with any technical person.
Also, a good logging framework makes the debugging very easy and failures can be identified very easily. Defect resolution decreases exponentially with logs. Logs tell about the health of the project to the stakeholders. So, you can imagine how important is to maintain a good logging framework.
Log4j - Logging Framework details
For now, we will be using as a Log4j logging framework. This framework is a JAVA-based API which is generally used by developers and testers to generate logs. It is open source so you would get it for free. You can download and use this API for logging purpose.
Now, let us dig more into the components of log4j. Let’s see what they are.
Loggers
It has all the information about the log level. Logs severity depends on the action being performed. So, different log levels are there which represents different log severity in a project.
The first step to use logger would be creating an object of Logger class.
Logger logger = Logger.getLogger("ClassName");
Let’s look at the severity levels which log4j provides. It has basically 5 kinds of severity log levels. Let’s look into details of these levels.
All: You can log almost everything with this type of log level.
DEBUG: You can print the debugging information with this log level and most developers will be using this log level.
log.debug("info”);
INFO: Whenever you want to print something related to the progress of an operation or a test, and then you should use this log level.
log.info("info");
WARN: Whenever you find some faulty and unexpected system behaviour, then you should use WARN log level.
ERROR: Whenever an error can be traced then you should use an ERROR log level.
FATAL: Whenever you find something which can crash the system, then you should use FATAL log level.
OFF: It disables the logging.
Appenders
Now, you have noted what will be the logs but what would happen to those logs that would be decided by appenders. It will be sending log events to the destination folder. There are basically three types of appenders. Let’s have a look at those first.
ConsoleAppender Logs is used for sending logs to standard output.
FileAppender Logs will help in sending logs to the destination folder.
Rolling File Appenders will help in defining log file size. After that size, if files, the new log file will be created and logs will be printed in that.
Layouts
It has different methods which allow formatting of logs. It formats the logging information in different formats. There are basically two methods which are available to get a logger object to define the layout of the logs.
Public static Logger getRootLogger()
Public static Logger getLogger(String name)
Steps to configure Log4j into your existing framework
We will configure the log4j by having DEBUG level for lofs and RollingFileAppennder for sending logs to the destination folder. Let’s see how root logger will be set up and configured. “logfiles.logs” is the filename on which all logs will be sent. Pattern layout would be selected for configuration purpose for the very first time.
Let’s look at the terms which are used above so that you can have a better understanding of those terms.
- File: This is actually the file name in which the logs will be printed.
- MaxFileSize: So, you have to define the maximum size of the log file so that after size gets completed, a new log file will be created.
- MaxBackupIndex: Maximum number of files which you want logger to backup.
- Layout: It does the formatting of the logs.
- Append: It can have true or false values. If it is false, a new file is created after a maximum size of the log file has been reached. If it is true, then a new file would not be created.
Steps to use Log4j with Selenium
First of all, create a JAVA project with name Log4jFramework
You have to import all the log4j jars. First, download them from the internet and then go to configure build path and then go to the libraries folder. Select all external jars and then select all the jar files. You can see the below shot for more details.
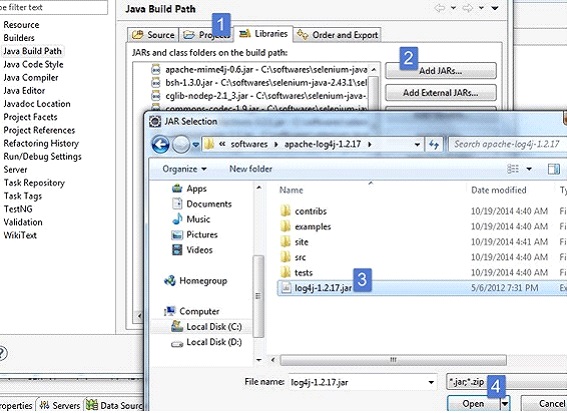
Now, what you have to do is to make a file name log4j.properties. You can create two more files one will be manual logs and one will be selenium logs in downloads folder.
After you are done with that, you have to add the following code in log4j.xml file you have just created:
Now, you have to create a java class in which you will actually call the logger instance and log the information. You can make a class name as log4jTest and then put the below coding in the file.
After you will run your tests, you will get the logs as below shot.
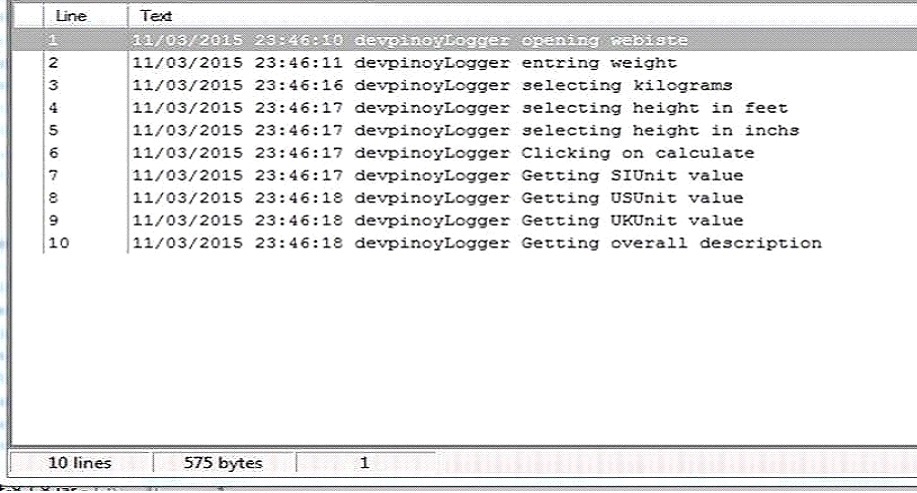
How to Debug with Logs
After you are done with tests execution, you can go through the logs which are generated. If at later point of times, some critical bug comes in and you have to look what went wrong and you want to go through the logs at the previous point of time, you can then go through the logs to find the root cause of the bug.
After the tests are run, you can quickly scan if there is ERROR or FATAL logs available. In this way, you will quickly resolve those severe issues. Also, logs are a common medium which can be shared with all stakeholders for more clarity.
Conclusion
So, with the help of log4j, you will be able to generate logs and hence, you will be able to debug the selenium scripts. So if you are providing Selenium Test Automation Services, then you can now easily debug the selenium scripts with the log4j logging framework. All the best!!
For more information visit here and get List of all Operations and Effective Elements of Selenium Automation Testing